Introduction
Futureverse is building the foundation for the next generation of immersive, decentralized experiences.
Our suite of tools and APIs empowers developers to build seamless, integrated applications that connect the digital and physical worlds. Whether you’re creating decentralized applications, integrating on-chain authentication, or building immersive experiences, Futureverse provides everything you need to succeed.
Why Futureverse?
At Futureverse, we aim to:
-
Simplify complex blockchain technology and infrastructure.
-
Innovate by offering tools that bridge physical and digital realms.
-
Empower developers with scalable, customizable APIs and SDKs.
Discover Futureverse
Futureverse’s comprehensive technology platform empowers developers to create the worlds they imagine. The Futureverse Platform features tools that combine the ease of web2 with the power and interoperability of web3.
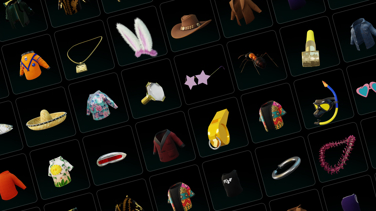
Assets
Fetch, filter, and display tokens and collectibles, enabling dynamic interactions in applications and gaming experiences.
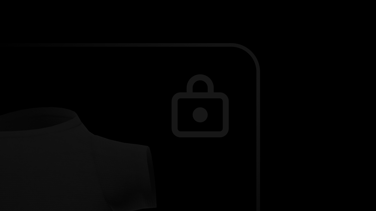
Transact
Create transactions like transfer and mint, with support for the signer SDK.
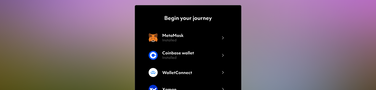
Auth
Simplify onboarding and data portability between different apps, games, and experiences.
Community and Support
Need help or have questions? Join our community, or reach out to our support team.